- Could Not Open Language File English.lng Dev C 2b 2b 1b
- Could Not Open Language File English.lng Dev C 2b 2b 1
- Could Not Open Language File English.lng Dev C 2b 2b C
Trying to run an executable file from Turbo C 13; Give a visual interface (GUI) to a console exe file 8; How can I create a game of tic-tac-toe using c 4; cant run exe files thar write in C 9; exe file size difference 3; Big calculator in Pascal 14; Converting the code from C to C 4; fixed point math in C 1. Libcitygml is a small and easy to use open source C library for parsing CityGML files in such a way that data can be easily exploited by 3D rendering applications (geometry data are tesselated and optimized for rendering during parsing).
Tags: C/C++, Programming, Raspberry PIRessources for Raspberry PI are generally written for Python but, as a micro computer, other languages can be used. If you know C/C++ ( If you come from the Arduino world, for instance) and don’t want to bother learning another computing language, it is possible to program Raspberry Pi using C/C++. In this tutorial we will see how to run C++ on Raspberry Pi and how to program your Raspberry Pi as an Arduino.
Material
- Raspberry Pi3 (or Zero) + monitor + keyboard
- Jumper cables F/F x2
- LED x1
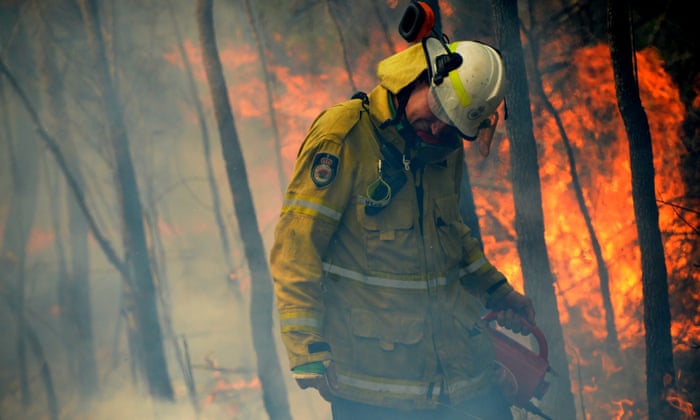
Geany
Raspbian is delivered with Geany which is a text editor that can be used to write and execute a Python code.
- Open Geany
- Create a new file
- In Documents > Define file type > Programming language > Select C or C++ source file
Copy the following code:
C++ program must be compiled before being executed. In the menu “Build” select “Build”, or press F9 directly, to compile the code. Then, in “Build” select “Execute”, or press F5, to run the code.
The phrase “Hello World” should be displayed in the terminal of the Raspberry PI.
Program your Raspberry Pi like an Arduino
Install WiringPi
To control the I/O of the Raspberry Pi like the ones of the microcontroller Arduino you can use the library wiringPi.h.
In a terminal, check that the library is installed by typing:
If an error occurs, update your Raspbian installation with the commands:
Then copy the GIT repository:
And compile the library:
WiringPi.h Wiring
The library WiringPi uses the GPIO numeration:
You can find the pinout by typing:
Base code to light a LED with Raspberry Pi
Once the library installed, you can write a program using the same keywords as the Arduino in addition to the main() function and the basic includes.
To compile the C++ program using the wiringPi library with Geany, in the Build>Set Build Commands, in the case corresponding to Build Command, type : g++ -Wall -o “%e” “%f” -lwiringPi. You can then compile the code using the “Build” button and run it correctly using key F5.
Warning: Arduino libraries , otherwise specified, are not supposed to be compatible with Raspberry Pi. You may have to write your own library.
How useful was this post?
Click on a star to rate it!
Average rating 4.8 / 5. Vote count: 6
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Let us improve this post!
Tell us how we can improve this post?
Could Not Open Language File English.lng Dev C 2b 2b 1b
What is C++?
- C++ is a general purpose programming language which has been used since the 1990’s
- It was designed by Bjarne Stroustrup under with the name “C with classes”.
- It is a version of C that includes Object-Oriented elements, including classes and functions.
- It is considered one of the biggest programming languages, as you can see in the following image:
source: Github
Your First Program in C++
The Output of this program will simply be :
Now, let’s break down the code:
Lines 1 and 2
- The first line tells the computer to use the “iostream” header file for this specific program . A header file is a seperate file with prewritten C++ code. There are many other header files which are requireed for a specific program to run properly. Some of them are : math , vector and string. Header files are generally represented by a “.h” extension (you don’t need to add .h when including C++ standard library files)
iostream
stands for input-output stream . The “iostream” file contains code for allowing the computer to take input and generate an output, using the C++ language.- The second line tells the computer to use the standard namespace which includes features of standard C++. You could write this program without this line, but you’d have to use
std::cout
instead ofcout
andstd::endl
instead ofendl
on line 4. It makes the code more readable and our lives as programmers easier.
Line 3 and 4
- C++ starts execution of a program from the -main function-
int main()
. During execution , the computer starts running the code from every line from{
(opening bracket) till}
(closing bracket) NOTE : Every function starts with an opening curly brace ”{” and ends with a closing curly brace ”}“. - Line 4 indicates the start of the main() function.
Lines 5, 6 & 7
- The word
cout
in C++ is used to output. - It is followed by
<<
, the insertion operator . - Whatever is in the double quotes
'
is printed . Certain special characters have a different syntax for print statements - Now to print any other kind of data , you have to add
<<
.
Challenge: Try to change Hello World to any other sentence or word(s). What will be the output ?
endl
is a reserved word when using the C++ language to end this line and go to the next line during output . - cout stands for “console output”- Finally, finish the command with a semicolon
;
.
NOTE : Every command except the main function definition and the #include directive needs to be ended by the semicolon. Without a ”;” , you may encounter an error.
return 0;
safely terminates the current function i.e. ‘main()’ in this case and since no function follows after ‘main()’ the program is terminated.- Don’t forget to tell the computer that this is end of the main() function. To do this , you add the closing curly brace ”}“. You will encounter an error before program execution if you do not include the } .
The code should look something like this:
Programmers use a Hello World program (like this one) as a ritual on using a new programming language. It is a symbol of good luck.
You have finished coding your first C++ program and have understood most of the code you have written/typed. CONGRATULATIONS!
Good Luck to all of you and happy coding! :)
Could Not Open Language File English.lng Dev C 2b 2b 1
Happy Coding ! :)
Feel free to ask any questions on FreeCodeCamp’s GitHub page or FreeCodeCamp’s Forum.
You may need some software to write and execute C++ code. I recommend using CodeBlocks. There’s a download link below :
Could Not Open Language File English.lng Dev C 2b 2b C
Download Link : Download Here
- Click the link with the GNU/GCC compiler for windows. This will not require an additional installation
Other alternatives could be visual studio, using a compiler or an online IDE such as Cloud9 or repl.it
Link #2 for Mac : Download for Mac #2 here